How can we safely upgrade the npm dependencies in our project? What do the funny ^
and ~
characters mean in front of the dependency package versions? How can we do a major version upgrade on an npm dependency in our project? We'll find out in this post.
Giving npm permission to install newer version. When executing npm install on a fresh checkout without existing nodemodules, npm downloads and installs a version that satisfies package.json for each dependency. Instead of specifying the exact version to be installed in package.json, npm allows you to widen the range of accepted versions. Here's the correct way to update dependencies using only npm from the command line. Updating to close-by version with npm update. When you run npm install on a fresh project, npm installs the latest versions satisfying the semantic versioning ranges defined in your package.json. After the initial install, re-running npm install does not update. Jan 10, 2019 The syntax can get complicated, depending on what you're trying to tell npm to do. This course limits examples to the syntax you're most likely to use. Example 1: Accept a single version only. Let's say you are not willing to tolerate any change to the current version of a dependency. In this case, you would specify just the version. Npm will look at package.json file and install all the dependencies according to their mentioned versions. This command is typically used when a Node project is forked and cloned. The nodemodules being a big folder is generally not pushed to a github repo and the cloner has to run npm install to install the dependencies. To manage these dependencies they utilize NPM which is a package manager for javascript oriented software. Moreover, this also applies to the backend as Node.js can be embedded in devices to act as the link between the web applications and the hardware.
Version parts
npm package versioning follows semantic versioning. So, a package version has 3 parts - Major.Minor.Patch
- Patch. This is incremented when a bug fix is made that won't break consuming code
- Minor. This is incremented when features are added that won't break consuming code
- Major. This is incremented when breaking changes are made
What does the ^
and ~
mean?
A version often has a ^
in front of it (e.g. ^16.8.6
). This means that the latest minor version can be safely installed. So in this example, ^16.12.1
can be safely installed if this was the newest version in 16.x
.
Sometimes a version has a ~
in front of it (e.g. ~16.8.6
). This means that only the latest patch version can be safely installed. So in this example, ^16.8.12
can be safely installed if this was the newest version in 16.8.x
.
So, npm install
installs the latest safe version of the dependencies?
Yes and no!
If the packages have already been installed into the node_modules
folder, then npm install
won't update any packages.
If the packages haven't been installed and a package-lock.json
file exists, then npm install
will install the exact dependency versions specified in package-lock.json
.
npm install
will install the latest safe version of the dependencies if they don't exist in the node_modules
folder and, there is no package-lock.json
file. However, you may think the latest safe version hasn't been installed because package.json
is unchanged, but if you check the packages in the node_modules
folder, the latest safe version will have been installed.
So, how do I safely update all the dependencies?
Npm Dependency Version Syntax Free
Firstly, the dependencies that are out of date can be discovered by running the following command:
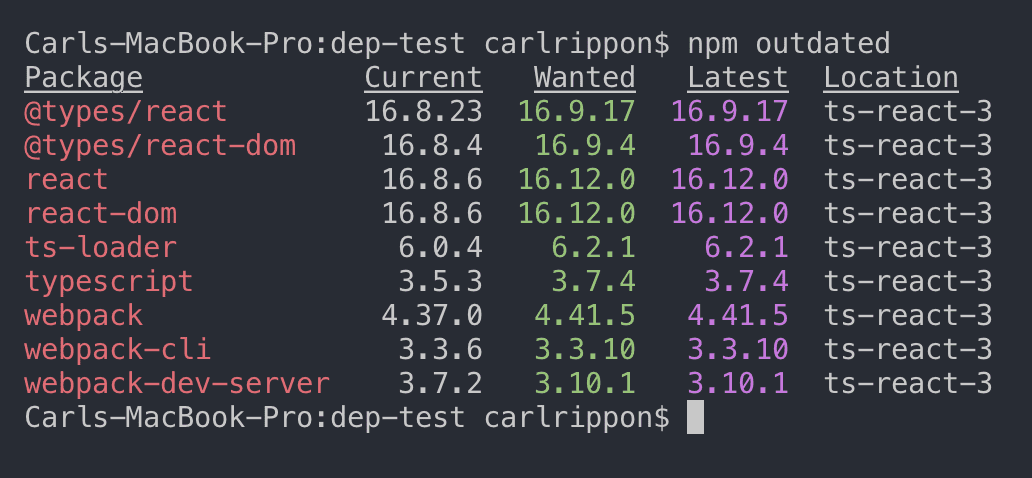
The dependencies will be listed out:
The wanted version is the latest safe version that can be taken (according to the semantic version and the ^
or ~
prefix). The latest version is the latest version available in the npm registry.
All the dependencies can be safely updated to the wanted version by using the following command:
As well as updating the packages in the node_modules
folder, the package.json
and package-lock.json
files will be updated.
If we don't want to update all of the packages, then the package names can be specified at the end of the command:
React is updated in the above example. King tuff was dead rar.
Updating all dependencies with major changes
So, how do we upgrade dependencies when there has been a major version change?
Perhaps the safest way is as follows:
- Check the changelog of the dependent package for breaking changes that could affect our app
- If we think we are safe to do the upgrade, run the following command:
- If multiple packages go together, you can list them all out. The example below will update React to the latest version:
- Verify the app isn't broken by doing some tests
- Repeat the process for other packages where there is a major version change
Is there a quicker way of just updating all the dependencies, including major version changes? So, like npm update
but for major version updates as well?
Yes, there is a tool called npm-check-updates that will do this. Just run the following command:
Winrar free download full version for windows 10. This will update the dependencies to the latest versions (including major version changes) in the package.json
file. If we are happy to go ahead with the upgrades we need to run the following command:
This will then upgrade the packages in the node_modules
folder, and the package-lock.json
file will be updated as well.
Wrap up
- Use
npm outdated
to discover dependencies that are out of date - Use
npm update
to perform safe dependency upgrades - Use
npm install @latest
to upgrade to the latest major version of a package - Use
npx npm-check-updates -u
andnpm install
to upgrade all dependencies to their latest major versions
Reposted from Domenic's blog with permission. Thanks!
npm is awesome as a package manager. In particular, it handles sub-dependencies very well: if my package depends onrequest
version 2 and some-other-library
, but some-other-library
depends on request
version 1, the resultingdependency graph looks like:
This is, generally, great: now some-other-library
has its own copy of request
v1 that it can use, while notinterfering with my package's v2 copy. Everyone's code works!
Npm Dependency Version Syntax
The Problem: Plugins
There's one use case where this falls down, however: plugins. A plugin package is meant to be used with another 'host'package, even though it does not always directly use the host package. There are many examples of this pattern in theNode.js package ecosystem already:
- Grunt plugins
- Chai plugins
- LevelUP plugins
- Express middleware
- Winston transports
Even if you're not familiar with any of those use cases, surely you recall 'jQuery plugins' from back when you were aclient-side developer: little </code>s you would drop into your page that would attach things to <code>jQuery.prototype</code>for your later convenience.</p><p>In essence, plugins are designed to be used with host packages. But more importantly, they're designed to be used with<em>particular versions</em> of host packages. For example, versions 1.x and 2.x of my <code>chai-as-promised</code> plugin work with<code>chai</code> version 0.5, whereas versions 3.x work with <code>chai</code> 1.x. Or, in the faster-paced and less-semver–friendly world ofGrunt plugins, version 0.3.1 of <code>grunt-contrib-stylus</code> works with <code>grunt</code> 0.4.0rc4, but breaks when used with <code>grunt</code>0.4.0rc5 due to removed APIs.</p><p>As a package manager, a large part of npm's job when installing your dependencies is managing their versions. But itsusual model, with a <code>'dependencies'</code> hash in <code>package.json</code>, clearly falls down for plugins. Most plugins never actuallydepend on their host package, i.e. grunt plugins never do <code>require('grunt')</code>, so even if plugins did put down their hostpackage as a dependency, the downloaded copy would never be used. So we'd be back to square one, with your applicationpossibly plugging in the plugin to a host package that it's incompatible with.</p><p>Even for plugins that do have such direct dependencies, probably due to the host package supplying utility APIs,specifying the dependency in the plugin's <code>package.json</code> would result in a dependency tree with multiple copies of thehost package—not what you want. For example, let's pretend that <code>winston-mail</code> 0.2.3 specified <code>'winston': '0.5.x'</code> inits <code>'dependencies'</code> hash, since that's the latest version it was tested against. <a href='https://torrentyo.mystrikingly.com/blog/how-to-pirate-photoshop-for-mac' title='How to pirate photoshop for mac'>How to pirate photoshop for mac</a>. As an app developer, you want thelatest and greatest stuff, so you look up the latest versions of <code>winston</code> and of <code>winston-mail</code>, putting them in your<code>package.json</code> as</p><h3>Npm Check Version</h3><p>But now, running <code>npm install</code> results in the unexpected dependency graph of</p><p>I'll leave the subtle failures that come from the plugin using a different Winston API than the main application toyour imagination.</p><h2>The Solution: Peer Dependencies</h2><p>What we need is a way of expressing these 'dependencies' between plugins and their host package. Some way of saying, 'Ionly work when plugged in to version 1.2.x of my host package, so if you install me, be sure that it's alongside acompatible host.' We call this relationship a <em>peer dependency</em>.</p><p>The peer dependency idea has been kicked around for literallyyears. Aftervolunteering to get this done 'over the weekend' ninemonths ago, I finally found a free weekend, and now peer dependencies are in npm!</p><p>Specifically, they were introduced in a rudimentary form in npm 1.2.0, and refined over the next few releases intosomething I'm actually happy with. Today Isaac packaged up npm 1.2.10 intoNode.js 0.8.19, so if you've installed the latest version ofNode, you should be ready to use peer dependencies!</p><p>As proof, I present you the results of trying to install <code>jitsu</code> 0.11.6 with npm1.2.10:</p><p>Online xbox <a href='https://herexup144.weebly.com/online-xbox-remote.html'>remote</a>. As you can see, <code>jitsu</code> depends on two Flatiron-related packages, which themselves peer-depend on conflicting versionsof Flatiron. Good thing npm was around to help us figure out this conflict, so it could be fixed in version 0.11.7!</p><h2>Using Peer Dependencies</h2><p>Peer dependencies are pretty simple to use. When writing a plugin, figure out what version of the host package youpeer-depend on, and add it to your <code>package.json</code>:</p><p>Now, when installing <code>chai-as-promised</code>, the <code>chai</code> package will come along with it. And if later you try to installanother Chai plugin that only works with 0.x versions of Chai, you'll get an error. Nice!</p><div><strong>UPDATE:</strong> npm versions 1 and 2 will automatically install peerDependencies if they are not explicitly depended upon higher in the dependency tree. For all following versions of npm (starting with [email protected]), this will no longer be the case. You will receive a warning that the peerDependency is not installed instead.</div><p>One piece of advice: peer dependency requirements, unlike those for regular dependencies, <em>should be lenient</em>. Youshould not lock your peer dependencies down to specific patch versions. It would be really annoying if one Chai pluginpeer-depended on Chai 1.4.1, while another depended on Chai 1.5.0, simply because the authors were lazy and didn't spendthe time figuring out the actual minimum version of Chai they are compatible with.</p><p>The best way to determine what your peer dependency requirements should be is to actually followsemver. Assume that only changes in the host package's major version will break your plugin. Thus,if you've worked with every 1.x version of the host package, use <code>'~1.0'</code> or <code>'1.x'</code> to express this. If you depend onfeatures introduced in 1.5.2, use <code>'>= 1.5.2 < 2'</code>.</p><p>Now go forth, and peer depend!</p><br><br><br><br>